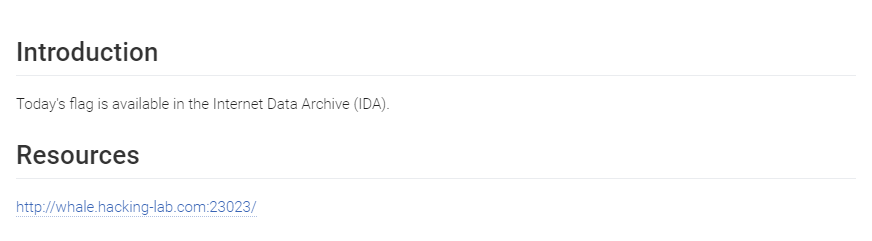
The page asks for a username and files to be downloaded. Dowloading the flag is not allowed.
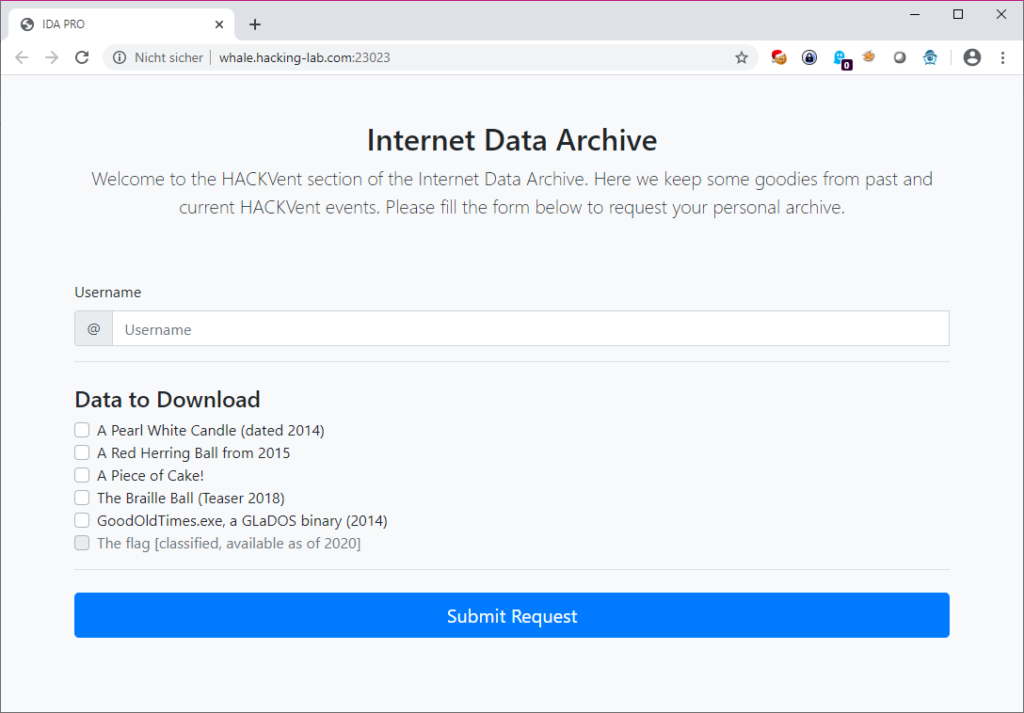
Submitting the form leads to another page with a download link and the password for the generated zip file. The generated files are all stored within the /tmp folder. Apache allows indexes on this folder so sorting the contents by last modified shows Santa-data.zip and phpinfo.php from October.
Santa’s archive is encrypted with an unknown password. The name of the website hints to the way IDA Pro installer passwords were generated before.
I wrote a small python script to get the used charset from some generated passwords.
data = ["az8aK9eeLCz8", "a97xqbLet7ST", "mXP5yai7XPhT", "8aSA2B7LA7ZL", "pm8S4T5QrJgX", "3pKeVidjKExs", "qtd9XZ6xdCL3", "RmpZigCseLZX", "RHmLVdjhRTsL", "BhE8bqC7bGem", "QRfq3ueKbmBV", "9zAWHBYcxa6S", "4VFCPc5MBJ5B", "ku65AbCDVeJW", "wpDuRYZ3Yu89", "sKy9ZubXcxjk", "LiEhhJE9Bcv9", "rAvtpSDxgPsP", "WS2zUAKKwBM7", "VfTD5EmETAeC", "8wMfAbmdM3vL", "eDekq2xswSHR", "8rdhRdpM9qcu", "p2g7XzcLcusF", "WsiAVmFQfRcy"] chrs = "" for s in data: for c in s: if not c in chrs: chrs += c print("".join(sorted(chrs))) print(len("".join(sorted(chrs))))
After that I wrote a small c++ program to generate a wordlist containing all passwords generated using the mt19937 algorithm (used by php) with rising seed.
#include <stdio.h> #include <stdlib.h> #include <string.h> #include <stdint.h> #include <random> #include <iostream> char* random_password(int seed, char* alpha) { char* pass = (char*) malloc(12 * sizeof(char)); std::mt19937 mt_rand(seed); for (int i = 0; i < 12; i++) { int n = mt_rand() % 54; *(pass + i) = *(alpha + n); } return pass; } int main() { char alphas[6][55] = { "23456789ABCDEFGHJKLMPQRSTUVWXYZabcdefghijkmpqrstuvwxyz", "23456789abcdefghijkmpqrstuvwxyzABCDEFGHJKLMPQRSTUVWXYZ", "ABCDEFGHJKLMPQRSTUVWXYZ23456789abcdefghijkmpqrstuvwxyz", "ABCDEFGHJKLMPQRSTUVWXYZabcdefghijkmpqrstuvwxyz23456789", "abcdefghijkmpqrstuvwxyz23456789ABCDEFGHJKLMPQRSTUVWXYZ", "abcdefghijkmpqrstuvwxyzABCDEFGHJKLMPQRSTUVWXYZ23456789" }; for (int i = 0; i <= 0xffffffff; i++) { for (int j = 0; j < 6; j++) { char* alpha = alphas[j]; char* pass = random_password(i, alpha); printf("%s\n", pass); } } }
After that i used zip2john to extract a hash for Santa’s zip archive and used john the ripper to bruteforce the hash using the wordlist just generated. After some time john returned Kwmq3Sqmc5sA as password for the zip file.
Decrypting the zip file and extracting the flag.txt returns the flag: HV19{Cr4ckin_Passw0rdz_like_IDA_Pr0}.